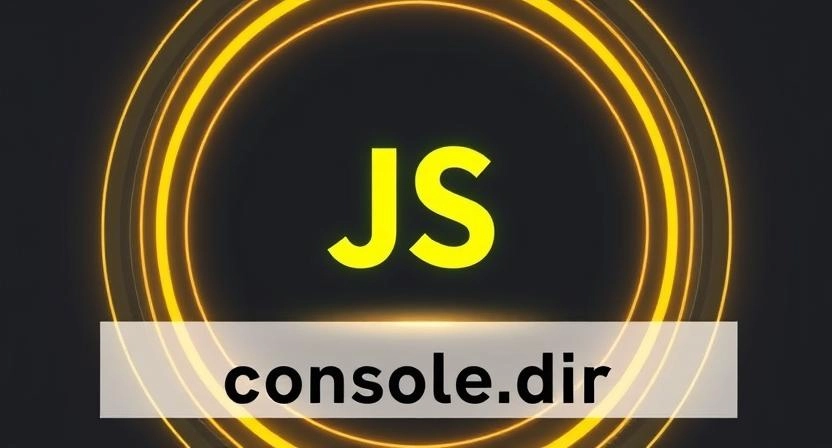
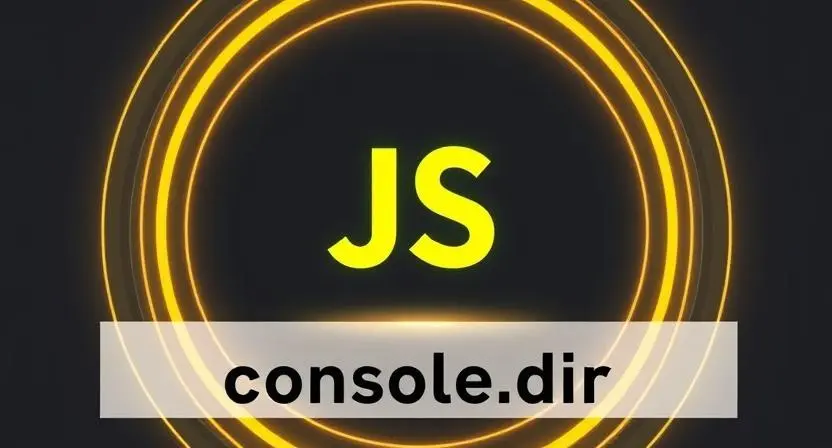
#CodeUtilize Explored: console.dir
In this article, we’ll walk through several methods of inspecting objects in JavaScript and TypeScript. We’ll start with the basic console.log
, then use console.log
combined with the util.inspect
method to get a deeper view of an object, proceed to using console.dir
which comes with its own built-in depth options, and finally compare console.dir
with console.log
. Let’s dive in!
Using -> console.log
The most straightforward way to print an object is by using console.log
. This method is ideal for quickly checking the contents of simple objects:
// Define a sample objectconst personAccount = { name: "Aldo", age: 20, cash: { account: { bank: { a: { a1: 1000000, a2: 2000000 } } } },};
// Displaying object structureconsole.log(personAccount);
// Output :// { name: 'Aldo', age: 20, cash: { account: { bank: [Object] } } }
While console.log provides a basic output, it might not display nested properties in the most navigable way when the objects become more complex.
Using -> console.log with util.inspect
For a more detailed view, especially when dealing with deeply nested objects, Node.js offers the util.inspect
function. This utility lets you define how deeply an object is rendered. Here’s an example:
const inspect = require("util").inspect;
// Define a sample objectconst personAccount = { name: "Aldo", age: 20, cash: { account: { bank: { a: { a1: 1000000, a2: 2000000 } } } },};
// Using util.inspect to view the full depth of the objectconsole.log(inspect(personAccount, { depth: Infinity, colors: true }));
// Output :// {// name: 'Aldo',// age: 20,// cash: {// account: { bank: { a: { a1: 1000000, a2: 2000000 } } }// }// }
In this snippet, passing { depth: Infinity }
ensures that every level of the object is printed, making it easier to inspect deeply nested properties.
To further explore the options and nuances of both console.log
and util.inspect
, consider checking out these resources: documentation
Using -> console.dir Directly
console.dir
is designed specifically for listing an object’s properties in an interactive, tree-like format without needing any additional utilities. It’s particularly handy for inspecting DOM elements or any large objects without extra configuration:
// Define a sample objectconst personAccount = { name: "Aldo", age: 20, cash: { account: { bank: { a: { a1: 1000000, a2: 2000000 } } } },};
// console.dir will output an expandable object tree in the consoleconsole.dir(personAccount, { depth: Infinity, colors: true });
// Output :// {// name: 'Aldo',// age: 20,// cash: {// account: { bank: { a: { a1: 1000000, a2: 2000000 } } }// }// }
Here, setting depth: Infinity
makes sure all nested levels are shown, much like util.inspect
, but with a focus on being interactively expandable in many browsers’ developer consoles.
console.dir vs console.log
Let’s take a moment to compare these two methods side-by-side:
console.log:
- Provides a quick, general output of an object.
- Best for simple or shallow objects.
- When used with deeply nested objects, the output can become hard to navigate.
console.dir:
- Specializes in displaying an object’s properties in a more organized, tree-like format.
- Supports options like depth and colors which enhance readability.
- Ideal for complex objects or debugging UI elements within the browser.
- By understanding the pros and cons of each, you can choose the correct method based on your debugging needs.
Conclusion
In summary, while console.log
is perfect for simple logging, combining it with util.inspect
or using console.dir
directly provides a much clearer insight into complex, nested objects. Whether you use util.inspect
or console.dir
might depend on your environment—Node.js versus browser debugging—but both methods empower you to gain finer control over how object data is viewed.
If you enjoyed this exploration of JavaScript's utilize methods
, be sure to check out my GitHub page for more projects, code examples, and development tips. Don’t forget to star or fork the repositories if you find them helpful. Happy debugging!!
← Back to blog