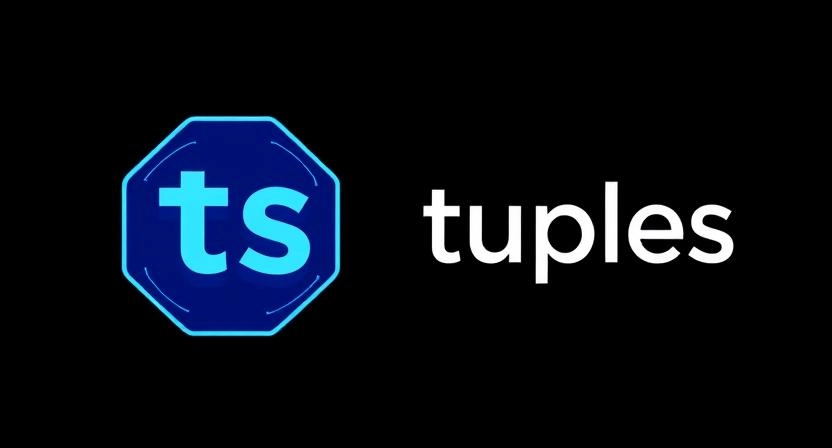
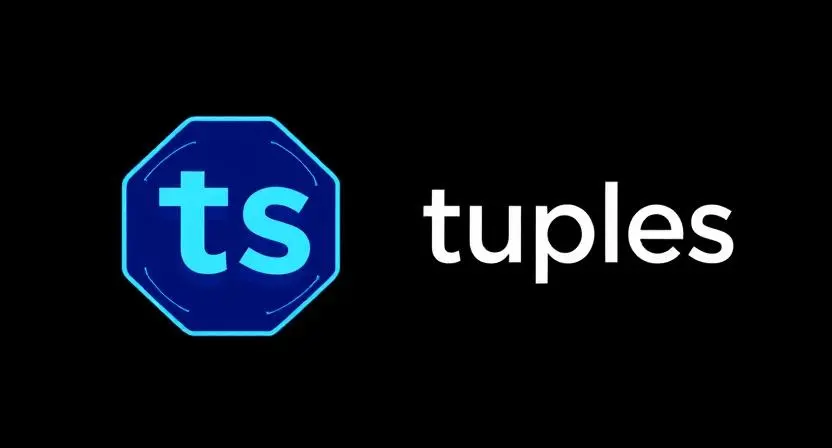
#LearnTypeScript: Demystifying Tuples
In this post, we explore one of the unique data types in TypeScript
: tuples. While arrays in JavaScript
are used to store collections of similar elements, tuples in TypeScript
allow you to store fixed-size collections of elements with known types at each index. Let’s dive into what tuples are, how to use them, and when they’re especially useful.
What Are ‘Tuples’ in TypeScript?
A tuple is a type that represents an array with a fixed number of elements where each element can be of a different type. For example, if you need a function that returns both a status code (a number) and a message (a string), you might use a tuple to enforce that structure:
// A simple tuple containing a number and a stringconst result: [number, string] = [200, "OK"];
This tuple ensures that the first element is always a number and the second a string. Tuples provide stricter
type checking compared to regular arrays.
How to Use Tuples
Declaring Tuples
Tuples are declared just like arrays, but you specify each element’s type in an exact order:
// Tuple representing a user's profile: [ID, username, isActive]const userProfile: [number, string, boolean] = [1, "Alice", true];
// Declare a tuple typetype MyTuple = [number, string, boolean];
// Create a tupleconst myTuple: MyTuple = [10, "Hello", true];
If you try to reorder or assign values of incorrect types, TypeScript
will throw an error, which helps catch potential bugs at compile time.
Accessing Tuple Elements
You can access tuple elements by their index as you would with arrays:
// Example 1 :const [userId, username, isActive] = userProfile;
console.log(userId); // Output: 1console.log(username); // Output: Aliceconsole.log(isActive); // Output: true
// Example 2 :type MyTuple = [number, string, boolean];const myTuple: MyTuple = [10, "Hello", true];
// Iterate over tuple elements with a loopfor (const element of myTuple) { console.log(element);}
Using Tuples in Functions
// A function returning a tuple with a status code and messagefunction fetchStatus(): [number, string] { // ... return [404, "Not Found"];}
const [code, message] = fetchStatus();
Using Tuples in Some Methods
type MyTuple = [number, string, boolean];const myTuple: MyTuple = [10, "Hello", true];
// Use methods like map, filter, and reduceconst mappedTuple: MyTuple = myTuple.map((element) => element * 2);console.log(mappedTuple); // Output: [20, "HelloHello", NaN]
const filteredTuple: MyTuple = myTuple.filter((element) => typeof element === "string");console.log(filteredTuple); // Output: [NaN, "Hello", NaN]
const reducedValue: number = myTuple.reduce((acc, curr) => acc + (typeof curr === "number" ? curr : 0), 0);console.log(reducedValue); // Output: 10
When to Use Tuples
Tuples are particularly useful in scenarios where you have a known and fixed
set of values with different types. Some common cases include:
Return values
: When functions return multiple values.Data modeling
: For representing a fixed structure, such as key-value pairs, with strict types.Configuration options
: When a function requires a fixed set of parameters.
Compared to arrays which are more flexible but less strict
, tuples provide better type safety and clarity in your code, ensuring that the data adheres to a specific structure.
Additional Resources
To further explore and deepen your understanding of tuples in TypeScript
, consider checking out these resources:
-
TypeScript Handbook - Tuple Types:
Explore the officialTypeScript
documentation onTuple Types
:
TypeScript Documentation: Tuple Types -
Tutorial on Tuples:
This blog post offers a comprehensive tutorial on tuples with practical examples:
Understanding TypeScript Tuples
Conclusion
Tuples in TypeScript
empower developers to enforce a strict structure where each element’s type is known and fixed.
They are ideal when functions need to return multiple values or when modeling data with mixed types.
With tuples, you can leverage TypeScript's
powerful type system to write safer and more maintainable code.
If you enjoyed this series of Learn TypeScript
, be sure to check out my GitHub page for more projects, code examples, and development tips. Don’t forget to star or fork the repositories if you find them helpful. Happy Coding!!
← Back to blog