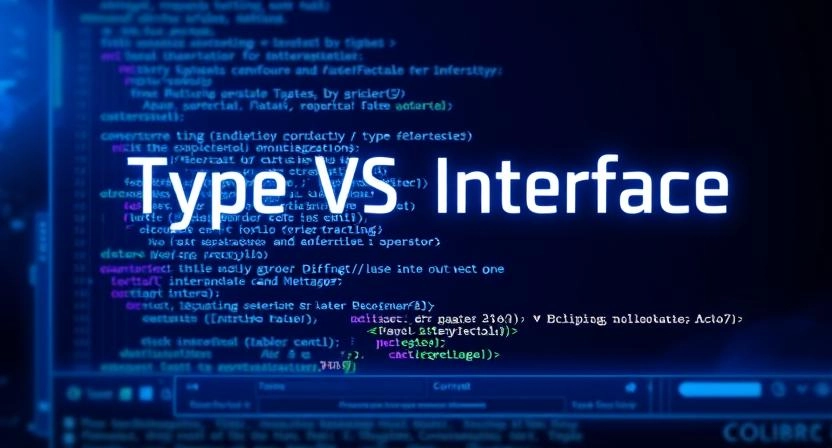
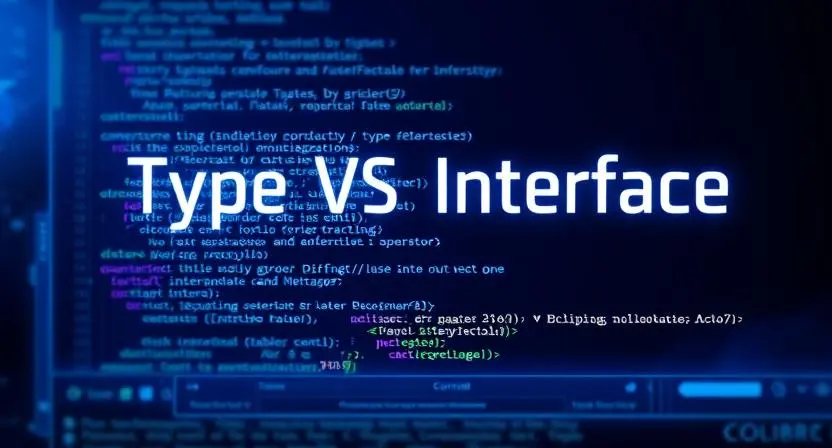
#LearnTypeScript: Type VS Interface
In this comprehensive guide, we dive deep into two of the most fundamental constructs in
TypeScript
—type aliases and interfaces. Whether you’re building a small application or working on an enterprise-level project,
understanding the subtle differences between these constructs is essential for robust type design. In this post, we will:
- Define type aliases and interfaces
- Provide practical code examples
- Compare their differences and typical use cases
- Discuss real-world scenarios and best practices
Let’s explore these elements step-by-step.
What Are Type Aliases?
A type alias in TypeScript
is a way to give a name to any type, including primitives, object types, union types, and intersections.
They serve as a tool to simplify complex type annotations and improve code readability.
Example: Object and Union Types
// Object Type Aliastype User = { id: number; name: string; isActive: boolean;};
// Using the type alias for an objectconst user: User = { id: 1, name: "Aldo", isActive: true,};
// Union Type Aliastype ID = number | string;
// Example usage of the union type aliasconst userId: ID = "12345"; // Can also be a number
In the examples above, type aliases provide a clear, reusable way to define the structure of data while ensuring that each component follows a consistent design.
What Are Interfaces?
An interface in TypeScript is specifically designed to define the shape of objects. Interfaces enable developers to declare contracts for object structures, promoting code consistency and clarity. They are particularly powerful in scenarios where you want to create hierarchical or extendable types.
Example: Basic Object Interface
// Defining an interface for a user objectinterface IUser { id: number; name: string; isActive: boolean;}
// Implementing the interfaceconst userInterface: IUser = { id: 1, name: "Aldo", isActive: true,};
Declaration Merging and Extension
One standout feature of interfaces is declaration merging. Multiple declarations of the same interface get automatically merged into one. This is especially valuable when extending external libraries or when the interface needs augmentation over time.
// First declaration of IUserinterface IUser { id: number; name: string; isActive: boolean;}
// Merging additional properties into IUserinterface IUser { email: string;}
// Now, IUser contains id, name, isActive, and email.const userWithEmail: IUser = { id: 2, name: "Ignata", isActive: false,};
Interfaces can also extend each other or even extend classes, allowing for greater flexibility
in building up complex data models.
interface IAddress { street: string; city: string;}
interface IUserProfile extends IUser { address: IAddress;}
const profile: IUserProfile = { id: 3, name: "Chandra", isActive: true, address: { street: "456 Elm St", city: "Surabaya", },};
Key Differences and Considerations
Declaration Merging
Interfaces
: Support declaration merging, which means you can declare an interface in multiple places and have them automatically combined.Type Aliases
: Do not support merging. Each type alias stands on its own.
Extensibility
Interfaces
: Are inherently designed to be extended. They can be extended by other interfaces or classes using the extends keyword.Type Aliases
: While you can form intersections (&) to create new composite types, they are less intuitive for hierarchical extension.
Use Cases
-
Interfaces
:- Ideal for defining the public contracts of a module or a class.
- Best when backward compatibility and augmentation might be needed (e.g., when third-party libraries extend your interfaces).
-
Type Aliases
:- Perfect for unions, intersections, and for aliasing primitives or other types.
- Useful when creating one-off types that do not require extension or merging.
Practical Considerations
When choosing between an interface
and a type alias
:
- If you need to extend or merge types, default to using interfaces.
- If you are, for example, creating complex union types or need a shorthand for composite types, type aliases can be more efficient and clearer.
Deep Dive: Real-World Scenarios
API Response Modeling
Imagine you’re modeling an API response for a user profile. If the API might add additional fields in the future or you want to allow third-party libraries to extend your type, using an interface is preferable.
interface ApiResponse { data: IUserProfile; status: number; message: string;}
Complex Data Structures
For complex logic involving state management, you might combine different types using unions or intersections. In these cases, type aliases shine.
type Action = | { type: "ADD_USER"; payload: User } | { type: "REMOVE_USER"; payload: number } | { type: "UPDATE_USER"; payload: User };
const performAction = (action: Action) => { // Handle actions};
Additional Resources
-
TypeScript Handbook - Basic Types:
Explore the official TypeScript documentation on basic types, including type aliases and unions.
TypeScript Documentation: Basic Types -
Interfaces:
Learn more about interfaces and their unique features in the TypeScript Handbook.
TypeScript Documentation: Interfaces
Conclusion
Understanding when to use type aliases
versus interfaces
is key to leveraging the full power of TypeScript’s type system.
Interfaces
offer robust features like declaration merging and extendability, making them ideal for defining contracts and building scalable applications.
On the other hand, type aliases
provide a flexible way to handle complex types and combinations, particularly useful in advanced type manipulations.
By mastering these concepts, you enhance your ability to write clear, maintainable, and type-safe code—a crucial skill for any serious developer.
If you enjoyed this series of Learn TypeScript
, be sure to check out my GitHub page for more projects, code examples, and development tips. Don’t forget to star or fork the repositories if you find them helpful. Happy Coding!!
← Back to blog